Deploy Token Wallet
In this section we will learn how to deploy a token wallet of an existing token root contract.
INFO
TON Solidity compiler allows specifying different parameters of the outbound internal message that is sent via external function call. Note, all external function calls are asynchronous, so callee function will be called after termination of the current transaction. value
, currencies
, bounce
or flag
options can be set. See <address>.transfer() where these options are described.
Note: if value
isn't set, then the default value is equal to 0.01 ever, or 10^7 nanoever. It's equal to 10_000 units of gas in workchain. If the callee function returns some value and marked as responsible
then callback
option must be set. This callback function will be called by another contract. Remote function will pass its return values as function arguments for the callback function. That's why types of return values of the callee function must be equal to function arguments of the callback function. If the function marked as responsible
then field answerId
appears in the list of input parameters of the function in *abi.json
file. answerId
is function id that will be called.
Step 1: Write Deployment Script
Now lets write the scripts to deploy a Token Wallet using locklift and previously written script stats.
INFO
Before we start to write our scripts we need to make sure that there is a file named 02-deploy-wallet.ts
in the script
folder in the project root.
Deploying the Token Wallet of an existing Token Root contract using the locklift tool can be achieved by utilizing the code sample provided below:
Using the everscale-inpage-provider
to deploy a token wallet is as easy as a piece of cake! All we need to do is call the deployWallet
function on the root contract, as explained below:
/* Deploying a token wallet for alice */
// deploying a token wallet using deployWallet method on the token root contract
await tokenRootContract.methods
.deployWallet({
answerId: 0,
walletOwner: aliceAccount.address,
deployWalletValue: locklift.utils.toNano('2'),
})
.send({
from: aliceAccount.address,
amount: locklift.utils.toNano('4'),
});
// Fetching the newly deployed token wallet address by calling the walletOf method on the token root
const walletAddress: Address = (
await tokenRootContract.methods
.walletOf({
answerId: 0,
walletOwner: aliceAccount.address,
})
.call({})
).value0;
console.log(`TIP3 Wallet deployed at: ${walletAddress.toString()}`);
import {
Address,
Contract,
Transaction,
} from 'everscale-inpage-provider';
import * as tip3Artifacts from 'tip3-docs-artifacts';
import { provider, providerAddress } from './useProvider';
async function main() {
// Preparing token root address
const tokenRootAddress: Address = new Address(
'<YOUR_TOKEN_ROOT_ADDRESS>'
);
// creating an instance of the token root contract
const tokenRootContract: Contract<
tip3Artifacts.FactorySource['TokenRoot']
> = new provider.Contract(
tip3Artifacts.factorySource['TokenRoot'],
tokenRootAddress
);
// Checking if the user already doesn't have any deployed wallet of that token root
const tokenWalletAddress: Address = (
await tokenRootContract.methods
.walletOf({ answerId: 0, walletOwner: providerAddress })
.call()
).value0;
// checking if the token wallet is already deployed or not
if (
(
await provider.getFullContractState({
address: tokenWalletAddress,
})
).state?.isDeployed
)
throw new Error(
'You already have a token wallet of this token !'
);
// Deploying a new token wallet contract
const deployWalletRes: Transaction = await tokenRootContract.methods
.deployWallet({
answerId: 0,
walletOwner: providerAddress,
deployWalletValue: 2 * 10 ** 9,
})
.send({
from: providerAddress,
amount: String(4 * 10 ** 9),
bounce: true,
});
// Checking if the token wallet is deployed
if (
(
await provider.getFullContractState({
address: tokenWalletAddress,
})
).state?.isDeployed
) {
console.log(
` Token wallet deployed to: ${(
await tokenRootContract.methods
.walletOf({ answerId: 0, walletOwner: providerAddress })
.call()
).value0.toString()}`
);
return true;
} else {
throw new Error(
`The token wallet deployment failed ! ${
(deployWalletRes.exitCode, deployWalletRes.resultCode)
}`
);
}
}
Step 2: Deploy Token Wallet
Use this command and deploy token wallet
npx locklift run -s ./scripts/02-deploy-wallet.ts -n local

Congratulations, you have deployed your first TIP3 Token Wallet 🎉
Token Root address
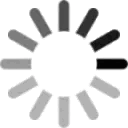