Mint TIP-3 Tokens
In previous section we have learned to deploy a token root and wallet using An account smart contract.
In this section we will learn the how to mint TIP-3 tokens for a token wallet.
To mint TIP-3 tokens we simply need to invoke the mint
function within the token root contract. Subsequently, the acceptMint
function will be triggered on the token wallet, resulting in the minting of tokens for the designated recipient token wallet.
Step 1: Write Minting Script
Utilize the code sample below to mint TIP-3 tokens using locklift tool and previously written script in the deploy token wallet section .
INFO
Before we start to write our scripts we need to make sure that there is a file named 03-mint-tip3.ts
in the script
folder in the project root.
The code sample below demonstrate minting TIP-3 tokens using everscale provider.
WARNING
Please be aware that if the notify
parameter is set to true for the transaction, the change will be sent back to the sender's token wallet
contract. Therefore, if you prefer to receive the change back into your account contract
, please ensure that the notify
checkbox remains unchecked.
/* Minting tip-3 token for bob */
// Preparing the parameters for minting tip-3 tokens
const mintAmount: number = 100;
let deployWalletValue: string = locklift.utils.toNano('2');
let txFee: string = locklift.utils.toNano('5');
// minting some token for the recipient
await tokenRootContract.methods
.mint({
amount: mintAmount * 10 ** decimals,
deployWalletValue: deployWalletValue,
notify: false,
recipient: bobAccount.address,
remainingGasTo: aliceAccount.address,
payload: '',
})
.send({
from: aliceAccount.address,
amount: txFee,
});
// Fetching the bobs balance
const bobTokenWallet: Contract<FactorySource['TokenWallet']> =
await locklift.factory.getDeployedContract(
'TokenWallet',
(
await tokenRootContract.methods
.walletOf({ answerId: 0, walletOwner: bobAccount.address })
.call()
).value0
);
const bobBal: number = Number(
(await bobTokenWallet.methods.balance({ answerId: 0 }).call())
.value0
);
console.log(`bob's ${symbol} balance: ${bobBal / 10 ** decimals}`);
import {
ProviderRpcClient as PRC,
Address,
Transaction,
Contract,
} from 'everscale-inpage-provider';
import * as tip3Artifacts from 'tip3-docs-artifacts';
import { provider, providerAddress } from './useProvider';
async function main() {
try {
// Required contracts Abi's
const tokenRootAddress: Address = new Address(
'<YOUR_TOKEN_ROOT_ADDRESS>'
);
const recipientAddress: Address = new Address(
'<RECIPIENT_ADDRESS>'
);
// Creating an instance of the token root contract
const tokenRootContract: Contract<
tip3Artifacts.FactorySource['TokenRoot']
> = new provider.Contract(
tip3Artifacts.factorySource.TokenRoot,
tokenRootAddress
);
// Fetching the token wallet address
const recipientTokenWalletAddress: Address = (
await tokenRootContract.methods
.walletOf({ answerId: 0, walletOwner: recipientAddress })
.call()
).value0;
// Fetching the decimals
const [decimals, symbol] = await Promise.all([
Number(
(
await tokenRootContract.methods
.decimals({ answerId: 0 })
.call()
).value0
),
(await tokenRootContract.methods.symbol({ answerId: 0 }).call())
.value0,
]);
// Defining the mint amount
const amount: number = 10 * 10 ** decimals;
// Checking if receiver has a wallet of this token root to specify the deployWalletValue parameter
let deployWalletValue: number = 0;
let txFee: number = 2 * 10 ** 9;
if (
!(
await provider.getFullContractState({
address: recipientTokenWalletAddress,
})
).state?.isDeployed
) {
deployWalletValue = 3 * 10 ** 9;
txFee = 5 * 10 ** 9;
}
// Deploying a new contract if didn't exist before
const mintRes: Transaction = await tokenRootContract.methods
.mint({
amount: amount * 10 ** decimals,
recipient: recipientAddress,
deployWalletValue: deployWalletValue,
remainingGasTo: providerAddress,
notify: false,
payload: '',
})
.send({
from: providerAddress,
amount: String(txFee),
});
if (mintRes.aborted) {
console.log(`Transaction aborted ! ${mintRes.exitCode}`);
return mintRes;
}
// Getting the recipient balance
const recipientTokenWalletContract: Contract<
tip3Artifacts.FactorySource['TokenWallet']
> = new provider.Contract(
tip3Artifacts.factorySource.TokenWallet,
recipientTokenWalletAddress
);
// Fetching the new balance of the receiver
const recipientBal: number =
Number(
(
await recipientTokenWalletContract.methods
.balance({ answerId: 0 })
.call({})
).value0
) /
10 ** decimals;
// Checking if the desired amount is minted for the receiver
if (recipientBal >= amount) {
console.log(
`${amount} ${symbol}'s successfully minted for recipient`
);
return `Hash: ${mintRes.id.hash} \n recipient ${symbol} \n balance: ${recipientBal}`;
} else {
console.log('Minting tokens failed !');
return `Failed \n
${(mintRes.exitCode, mintRes.resultCode)}`;
}
} catch (e: any) {
console.log(e.message);
return 'Failed';
}
}
Step 2: Mint TIP-3 tokens
Use this command to mint TIP-3 tokens:
npx locklift run -s ./scripts/03-mint-tip3.ts -n local
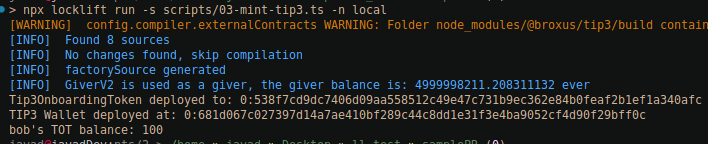
Congratulations, you have successfully minted TIP-3 tokens for a token wallet 🎉
INFO
In order to be able to mint token for a token wallet you must be the token root owner .
Token Root address
Recipient address
Amount